Summary
=======
Step 1) Start the AdminServer and NodeManager
Step 2) Login to weblogic admin console and start the managed server JMSTestMS
Step 3) Create persistence store
Step 4) Create a JMS Server
Step 5) Configuring JMS Module
Step 6) Creating Connection Factory
Step 7) Creating JMS Queue
Step 8) Create java file QueueSend.java
Step 9) Create java file QueueReceive.java
Step 10) Compile both files QueueSend.java and QueueReceive.java
Step 11) Send messages using QueueSend.java
Step 12) Receive messages using QueueReceive.java
Step 2) Login to weblogic admin console and start the managed server JMSTestMS
Step 3) Create persistence store
Step 4) Create a JMS Server
Step 5) Configuring JMS Module
Step 6) Creating Connection Factory
Step 7) Creating JMS Queue
Step 8) Create java file QueueSend.java
Step 9) Create java file QueueReceive.java
Step 10) Compile both files QueueSend.java and QueueReceive.java
Step 11) Send messages using QueueSend.java
Step 12) Receive messages using QueueReceive.java
Step 1) Start the AdminServer and NodeManager using below commands
cd /oracle/Middleware/user_projects/domains/prod_domain/bin
nohup ./startWebLogic.sh &
nohup ./startNodeManager.sh &

Step 2) Login to weblogic admin console and start the managed server JMSTestMS

Step 3) Create persistence store
Click on Services ?Click on Persistent Stores ?Click on New ? Select “Crete File Store”
Click on Services à Click on Persistent Stores à Click on New à Select “Crete File Store”

Name*: JMSFileStore
Target*:JMSTestMS
Directory: /oracle/Middleware/user_projects/domains/prod_domain

Click on OK

Step 4) Create a JMS Server
Click on Services à Click on Messaging àClick on JMS server

Click on New and Enter below details and click on Next
*Name:JMSServer
Persistent Store: select JMSFileStore

Select targets:JMSTestMS à Click on Finish


Step 5) Configuring JMS Module
Click on Services à Click on Messaging à Click on JMS Modules

Click on New and Enter below details à Click on Next
*Name: JMSSystemModule

Select Targets:JMSTestMS à Click on Next

Click on Finish


Step 6) Creating Connection Factory
Click on Services à Click on Messaging à Click on JMS Modules à Click on JMSSystemModule

Click on New

Select Connection Factory à Click on Next

Enter Below details à Click on Next
*Name: JMSConnectionFactory
JNDI: QJMSConnectionFactory

*Targets: JMSTestMS à Click on Finish


Step 7) Creating JMS Queue
Click on Services à Click on Messaging à Click on JMS Modules à Click on JMSSystemModule
Click on New

Select Queue à Click on Next

Enter below details and click on Next
Name: JMSQueue
JNDI Name: JMSQueue

Click on “Create New Subdeployment”
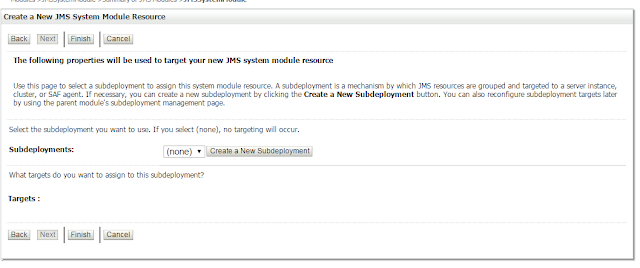
Enter Subdeployment name: JMSSubdeployment à Click on OK

Select Target : JMSServer à Click on Finish

Click on Finish
Step 8) Create java file QueueSend.java with following program
cd /oracle/Middleware/wlserver/server/bin
vi QueueSend.java
import
java.io.*;
import
java.util.Hashtable;
import
javax.jms.JMSException;
import
javax.jms.Queue;
import
javax.jms.QueueConnection;
import
javax.jms.QueueConnectionFactory;
import
javax.jms.QueueSender;
import
javax.jms.QueueSession;
import
javax.jms.Session;
import
javax.jms.TextMessage;
import
javax.naming.Context;
import
javax.naming.InitialContext;
import
javax.naming.NamingException;
public
class QueueSend
{
public
final static String JNDI_FACTORY="weblogic.jndi.WLInitialContextFactory";
public
final static String JMS_FACTORY="QJMSConnectionFactory";
public
final static String QUEUE="JMSQueue";
private
QueueConnectionFactory qconFactory;
private
QueueConnection qcon;
private
QueueSession qsession;
private
QueueSender qsender;
private
Queue queue;
private
TextMessage msg;
public
void init(Context ctx, String queueName)
throws
NamingException, JMSException
{
qconFactory
= (QueueConnectionFactory) ctx.lookup(JMS_FACTORY);
qcon
= qconFactory.createQueueConnection();
qsession
= qcon.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
queue
= (Queue) ctx.lookup(queueName);
qsender
= qsession.createSender(queue);
msg
= qsession.createTextMessage();
qcon.start();
}
public
void send(String message) throws JMSException {
msg.setText(message);
qsender.send(msg);
}
public
void close() throws JMSException {
qsender.close();
qsession.close();
qcon.close();
}
public
static void main(String[] args) throws Exception {
if
(args.length != 1) {
System.out.println("Usage:
java QueueSend WebLogicURL");
return;
}
InitialContext
ic = getInitialContext(args[0]);
QueueSend
qs = new QueueSend();
qs.init(ic,
QUEUE);
readAndSend(qs);
qs.close();
}
private
static void readAndSend(QueueSend qs) throws IOException, JMSException
{
String
line="Test Message Body with counter = ";
BufferedReader
br=new BufferedReader(new InputStreamReader(System.in));
boolean
readFlag=true;
System.out.println("\n\tStart
Sending Messages (Enter QUIT to Stop):\n");
while(readFlag)
{
System.out.print("<Msg_Sender>
");
String
msg=br.readLine();
if(msg.equals("QUIT")
|| msg.equals("quit"))
{
qs.send(msg);
System.exit(0);
}
qs.send(msg);
System.out.println();
}
br.close();
}
private
static InitialContext getInitialContext(String url) throws NamingException
{
Hashtable
env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY,
JNDI_FACTORY);
env.put(Context.PROVIDER_URL,
url);
return
new InitialContext(env);
}
}
Step 9) Create java file QueueReceive.java with following program
cd /oracle/Middleware/wlserver/server/bin
vi QueueReceive.java
import
java.util.Hashtable;
import
javax.jms.*;
import
javax.naming.Context;
import
javax.naming.InitialContext;
import
javax.naming.NamingException;
public
class QueueReceive implements MessageListener
{
public
final static String
JNDI_FACTORY="weblogic.jndi.WLInitialContextFactory";
public
final static String JMS_FACTORY="QJMSConnectionFactory";
public
final static String QUEUE="JMSQueue";
private
QueueConnectionFactory qconFactory;
private
QueueConnection qcon;
private
QueueSession qsession;
private
QueueReceiver qreceiver;
private
Queue queue;
private
boolean quit = false;
public
void onMessage(Message msg)
{
try
{
String
msgText;
if
(msg instanceof TextMessage)
{
msgText
= ((TextMessage)msg).getText();
}
else
{
msgText
= msg.toString();
}
System.out.println("\n\t<Msg_Receiver>
"+ msgText );
if
(msgText.equalsIgnoreCase("quit"))
{
synchronized(this)
{
quit
= true;
this.notifyAll();
// Notify main thread to quit
}
}
}
catch
(JMSException jmse)
{
jmse.printStackTrace();
}
}
public
void init(Context ctx, String queueName) throws NamingException, JMSException
{
qconFactory
= (QueueConnectionFactory) ctx.lookup(JMS_FACTORY);
qcon
= qconFactory.createQueueConnection();
qsession
= qcon.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
queue
= (Queue) ctx.lookup(queueName);
qreceiver
= qsession.createReceiver(queue);
qreceiver.setMessageListener(this);
qcon.start();
}
public
void close()throws JMSException
{
qreceiver.close();
qsession.close();
qcon.close();
}
public
static void main(String[] args) throws Exception
{
if
(args.length != 1)
{
System.out.println("Usage:
java QueueReceive WebLogicURL");
return;
}
InitialContext
ic = getInitialContext(args[0]);
QueueReceive
qr = new QueueReceive();
qr.init(ic,
QUEUE);
System.out.println("JMS
Ready To Receive Messages (To quit, send a \"quit\" message from
QueueSender.class).");
//
Wait until a "quit" message has been received.
synchronized(qr)
{
while
(! qr.quit)
{
try
{
qr.wait();
}
catch
(InterruptedException ie)
{}
}
}
qr.close();
}
private
static InitialContext getInitialContext(String url) throws NamingException
{
Hashtable
env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY,
JNDI_FACTORY);
env.put(Context.PROVIDER_URL,
url);
return
new InitialContext(env);
}
}
Step 10) Compile both files QueueSend.java and QueueReceive.java
cd /oracle/Middleware/wlserver/server/bin
. ./setWLSEnv.sh
javac QueueSend.java
javac QueueReceive.java

Step 11) Use the below URL for sending messages and enter any message for sending
[oracle@jagannathm bin]$cd /oracle/Middleware/wlserver/server/bin
[oracle@jagannathm bin]$. ./setWLSEnv.sh
[oracle@jagannathm
bin]$ java QueueSend t3://192.168.145.128:8003
Start Sending Messages (Enter QUIT to
Stop):
<Msg_Sender>
Hello World How Are you?

Step 12) Open another Putty session and enter below URL for receiving messages
[oracle@jagannathm bin]$cd
/oracle/Middleware/wlserver/server/bin
[oracle@jagannathm bin]$.
./setWLSEnv.sh
[oracle@jagannathm bin]$java
QueueReceive t3://192.168.145.128:8003
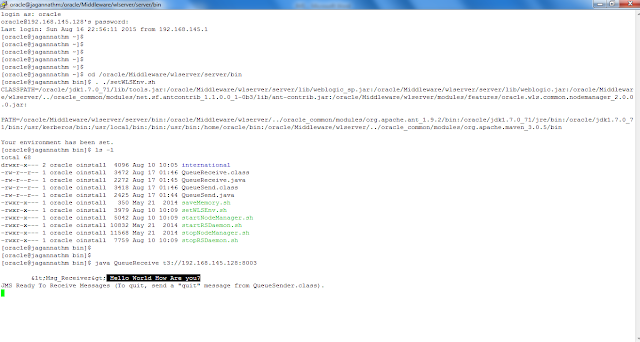
No comments:
Post a Comment